Amend your git commits
MP 114: Now is the best time to fix an unclear or misleading commit message.
Note: I will wrap up the Django from first principles series in the next few weeks. The next step is deployment, and that's a complicated enough topic that it will take a little while to write it up well.
It's safe to say that most Python developers use Git on a regular basis. If you're anything like me, you sometimes write a commit message only to realize it was a little unclear or misleading immediately after pressing Enter.
I've always known you can amend commit messages, but until recently I haven't made a habit of doing so unless they're egregiously bad. It's easy enough if you know the routine, so after a couple weeks of pushing myself to use git commit --amend
whenever I had that feeling after making a commit, it's become a good habit.
Writing a trading bot
I try to play with different kinds of data on a regular basis. I've always had a passing interest in finance, and when I was young I had a short-lived epiphany where I thought I could just write a program that would automatically buy stocks that are dropping, and sell them as soon as they go high again. I wrote my plans in a journal, fell asleep to dreams of getting rich, and then woke up to the realization that my program would quickly end up holding a basket of stocks that were never going to go back up.
I'm not the first to go through that cycle of excitement and disappointment about building a trading bot, and I won't be the last. When I first had that idea, retail investors didn't have access to platforms with APIs. Back then you'd have to be a professional broker at a computer-focused brokerage in order to even try writing a trading bot. But with the availability of "paper" accounts on platforms like Alpaca, I decided to give it a try. (Paper accounts let you write an algorithm that runs against current market data, in a sandboxed environment with fake funds.)
I don't want to be a day trader
There are a bunch of rules you need to be aware of if you're going to start trading algorithmically. One rule that can affect a paper trading account is the Pattern Day Trader rule. Basically, if I understand it right, the government doesn't want people engaging in day trading with small accounts. It's a highly risky activity, so not everyone should engage in day trading.
The rules are fairly simple: if you make four or more "day trades" within five business days, you're flagged as a day trader. If you have less than $25,000 in your account, you won't be able to trade until your day trade count drops. There's a bit more to it, but that's enough for the context of this post.
Here's my code to check whether a transaction would mark me as a pattern day trader:
def sell(asset): """Make a paper-trading sell.""" # Check if day trade limit will prevent sale. if (account.daytrade_count >= 3) and (account.equity < 25_000.00): msg = f"At daytrade limit, can't sell {asset}." ...
If I've already made three transactions that count as day trades, and I have less than $25,000 in the account, I can't make this transaction.
The "bad" commit message
This was the commit message I wrote just after making that change:
$ git commit -am "Checks day trade count before selling."
That seemed reasonable as I wrote it. But as I thought a little more about what counts as pattern day trading, and the code I wrote, I realized this was a little misleading. Basically, you can have more than three day trades if you have over $25,000 in your account. So I wasn't just checking the day trade count. This is a significant difference if I want my bot to behave appropriately with account values both below and above $25,000.
A better commit message
Without getting overly specific, I realized a simple change would make a much better entry in my Git log:
Checks day trade status before selling.
I reminded myself that amending a commit message is pretty simple, if you do it right away. You enter git commit --amend
, an editor opens with your existing message, you make your changes, and the new message is saved.
Here's what that process looks like:
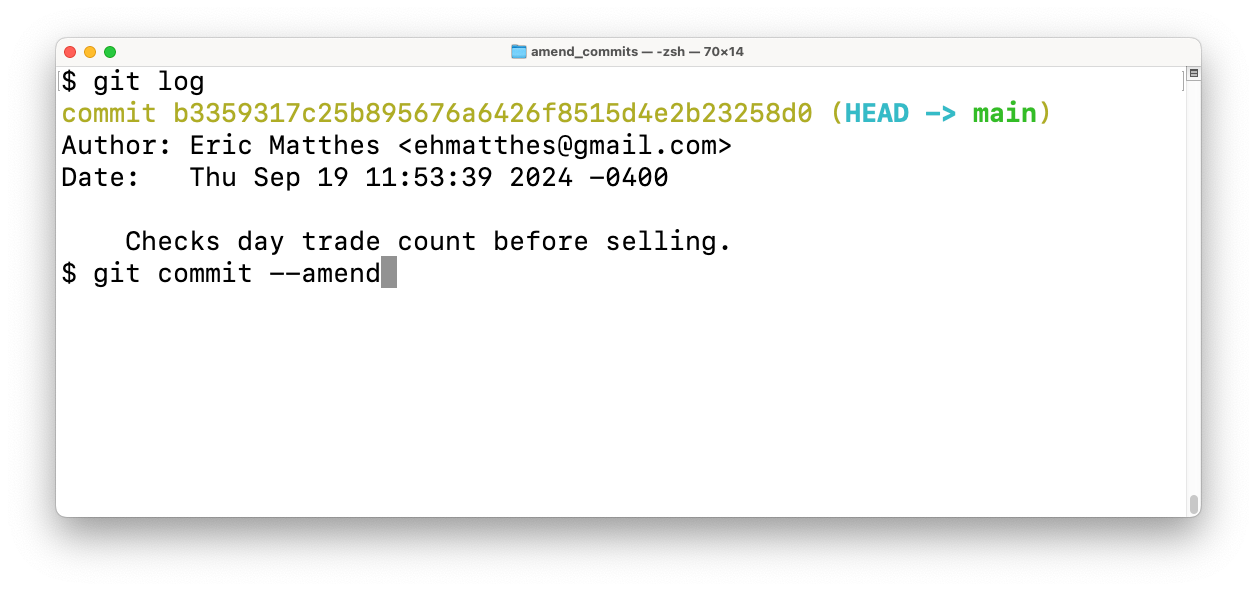
Looking at the log shows the original message, about checking the "day trade count".
After issuing the git commit --amend
command, an editor pops up:
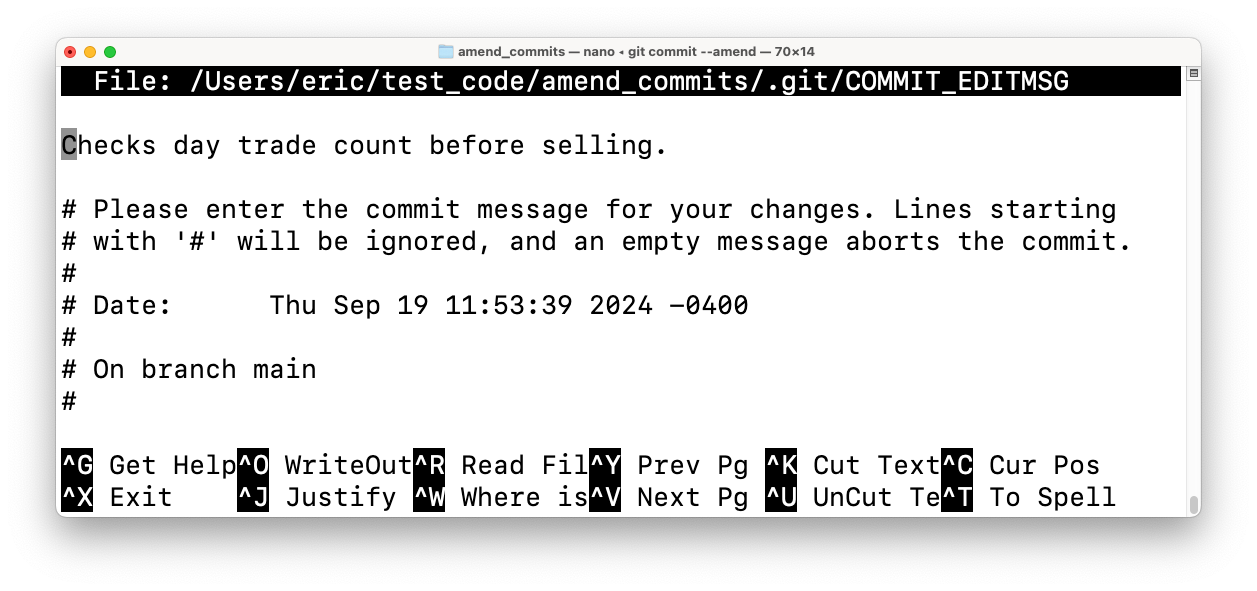
On my system, I have nano set as the default editor. The first line, which is the only uncommented line, is the original message ready to be edited.
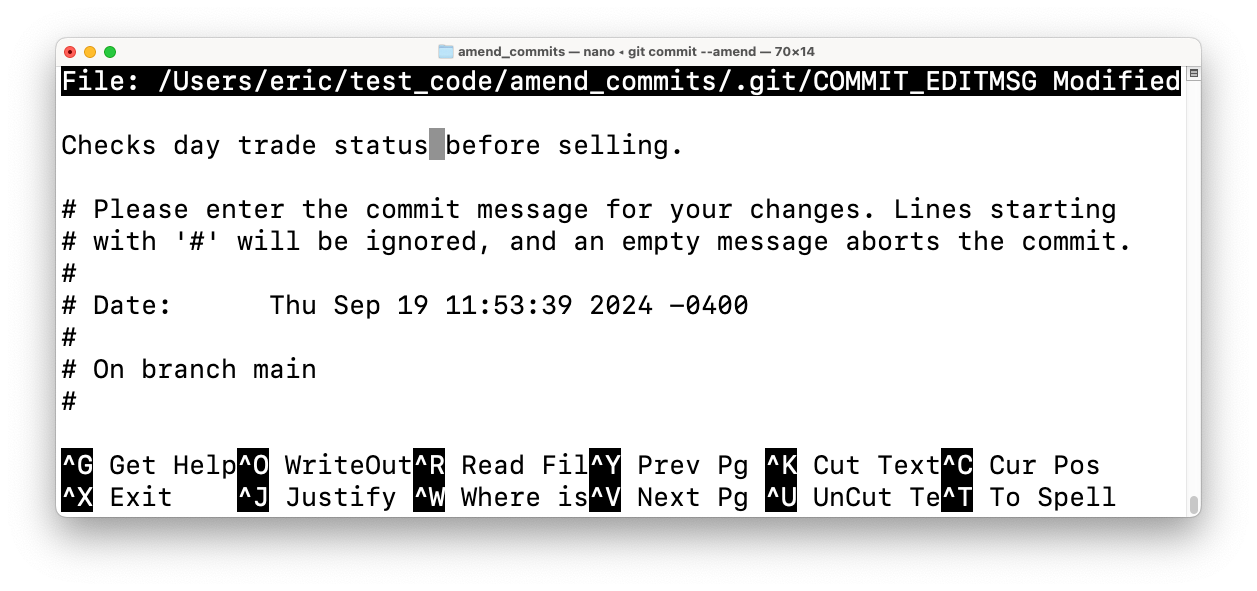
I've changed the message to mention checking the "day trade status", and it's ready to be saved.
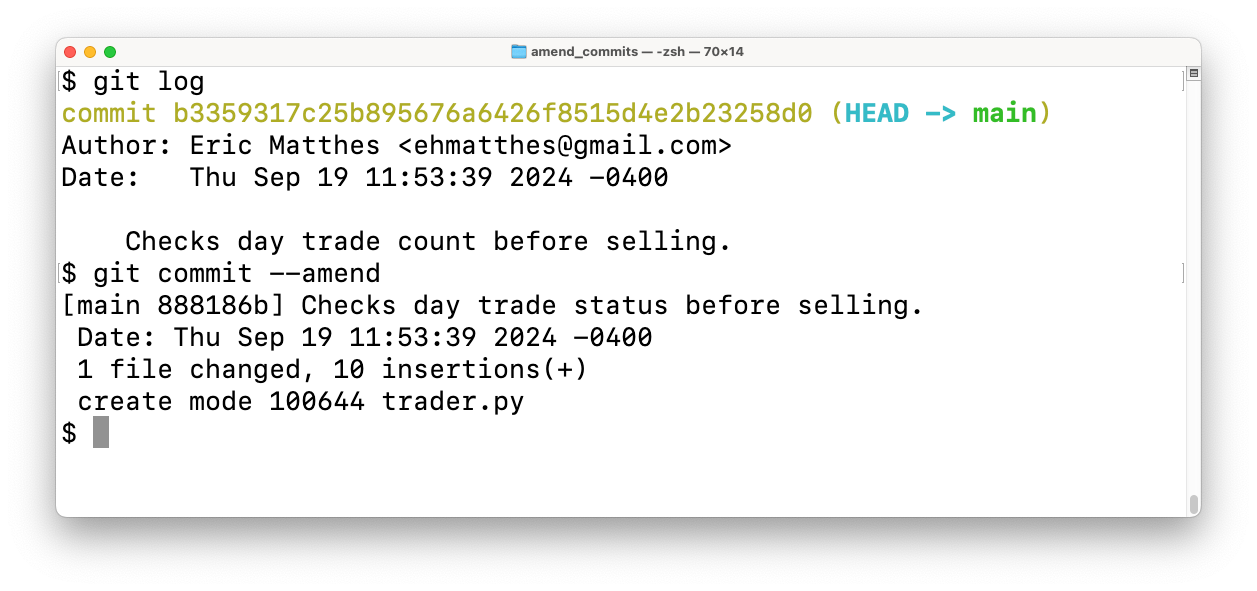
Saving and closing out of the editor shows that the message has been changed.
Running git log
again shows this change more clearly:
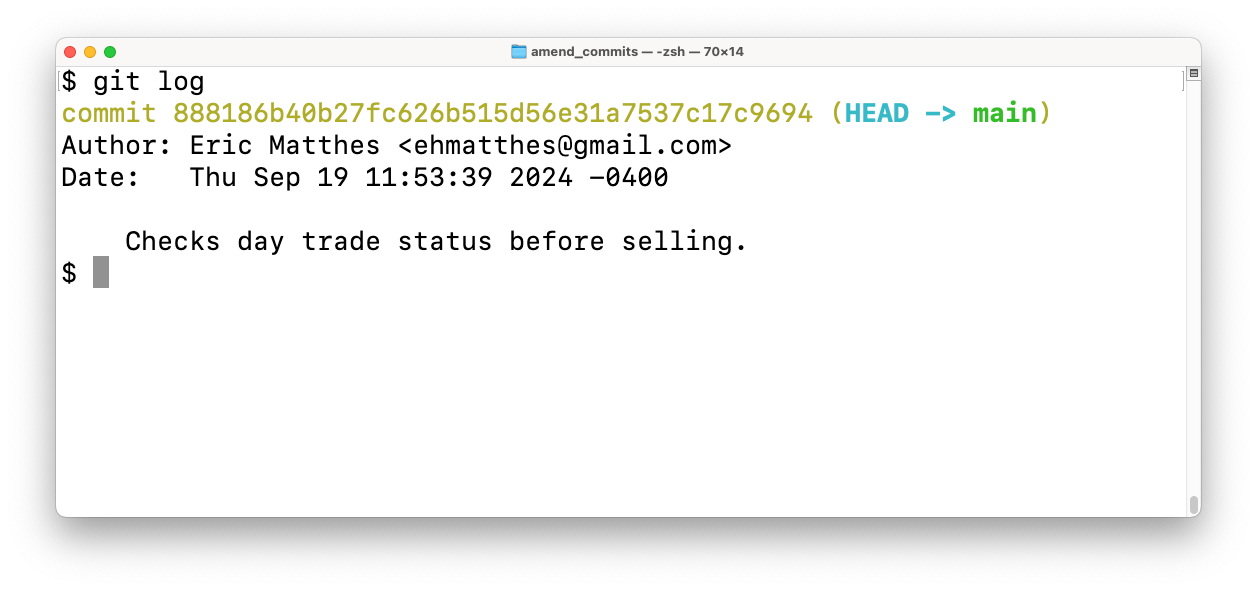
If you look at the commit hashes, you can see that the original commit has actually been replaced by a new one with the updated message. This is part of why it's better to use --amend
immediately after making a commit, before any new changes have been made.
Conclusions
Modifying your commits can be really complicated if they've been pushed to a repository, if the modification involves additional changes to the codebase, or if new changes have been made on top of the commit in question.
But if all you want to do is clarify a message on the commit you just made, make a habit of doing so right away. Your projects will be easier for you and others to follow, and you'll have one less nagging voice inside your head.